Shared Mode Master Client
Overview
The SharedModeMasterClient
is role given to one client in a Shared Mode
session. A player with this role will have a few unique privileges that other clients will not have access to.
Assignment
By default, the role of SharedModeMasterClient
will be assigned to the player who created a Shared Mode session. Players can determine if they are the SharedModeMasterClient
by simply calling NetworkRunner.IsSharedModeMasterClient
. This role will be reassigned if...
- The
SharedModeMasterClient
leaves or is disconnected; a newSharedModeMasterClient
will be automatically assigned to one of the clients currently in the room. - The
SharedModeMasterClient
executesNetworkRunner.SetMasterClient(PlayerRef player)
SharedModeMasterClient Privileges
As previously mentioned, the SharedModeMasterClient
has a few unique privileges.
Scene Management
In Shared Mode, only the SharedModeMasterClient
has the authority to manage scenes; this includes any method involved with scene management such as NetworkRunner.LoadScene
.
Initial State Authority over Scene Objects
When a new scene is loaded, the SharedModeMasterClient
will have StateAuthority
over all NetworkObjects
baked into the scene.
Is Master Client Object
In the Shared Mode Settings
for NetworkObjects
, if Is Master Client
is set to true, the SharedModeMasterClient
will always have State Authority over this NetworkObject. If a new SharedModeMasterClient
is assigned, the State Authority for this NetworkObject
will transfer to the new SharedModeMasterClient
.
Despawning Objects With No State Authority
If a NetworkObject
has Allow State Authority Override
checked and Destroy When State Authority Leaves
unchecked in its Shared Mode Settings
, the NetworkObject
will not be destroyed if the player disconnects; however, the SharedModeMasterClient
can despawn this object despite not having StateAuthority
over it.
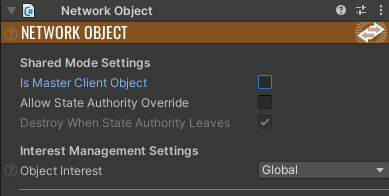
Tracking the Shared Mode Master Client
NetworkRunner.IsSharedModeMasterClient
will tell the user if they are the SharedModeMasterClient
but there is no built-in solution for letting other clients know who the SharedModeMasterClient
is. This can sometimes be useful in applications where letting the other players know who the SharedModeMasterClient in a session is. The following is one way this can be achieved:
- Create a
NetworkObject
and setIs Master Client Object
to true under theShared Mode Settings
. - Create a script like the following that can be used to send who the
SharedModeMasterClient
is and add it to the previously createdNetworkObject
:
using Fusion;
public class SharedModeMasterClientTracker : NetworkBehaviour
{
static SharedModeMasterClientTracker LocalInstance;
public override void Spawned()
{
LocalInstance = this;
}
private void OnDestroy()
{
if (LocalInstance == this)
LocalInstance = null;
}
public static bool IsPlayerSharedModeMasterClient(PlayerRef player)
{
if (LocalInstance == null)
return false;
return LocalInstance.Object.StateAuthority == player;
}
public static PlayerRef? GetSharedModeMasterClientPlayerRef()
{
if (LocalInstance == null)
return null;
return LocalInstance.Object.StateAuthority;
}
}
By storing a static instance of this NetworkBehaviour, users can then check to see which player is the SharedModeMasterClient
and respond accordingly.