Fusion 2 Introduction
Overview
For a list of changes from Fusion 1.1 to 2.0 check this page.
Fusion is a new high performance state synchronization networking library for Unity. Fusion is built with simplicity in mind to integrate naturally into the common Unity workflow, while also offering advanced features like data compression, client-side prediction and lag compensation out of the box.
Under the hood, Fusion relies on a state-of-the-art compression algorithm to reduce bandwidth requirements with minimal CPU overhead. Data is transferred as partial chunks with eventual consistency. A fully configurable area-of-interest system is supplied to allow support for very high player counts.
The Fusion API is designed to be similar to regular Unity MonoBehaviour code. For example, RPCs and network state is defined with attributes on methods and properties of MonoBehaviours with no need for explicit serialization code, and network objects can be defined as prefabs using all of Unity's most recent prefab features like nesting and variants.
Inputs, Networked Properties and RPCs provide the foundation for writing gameplay code with Fusion.
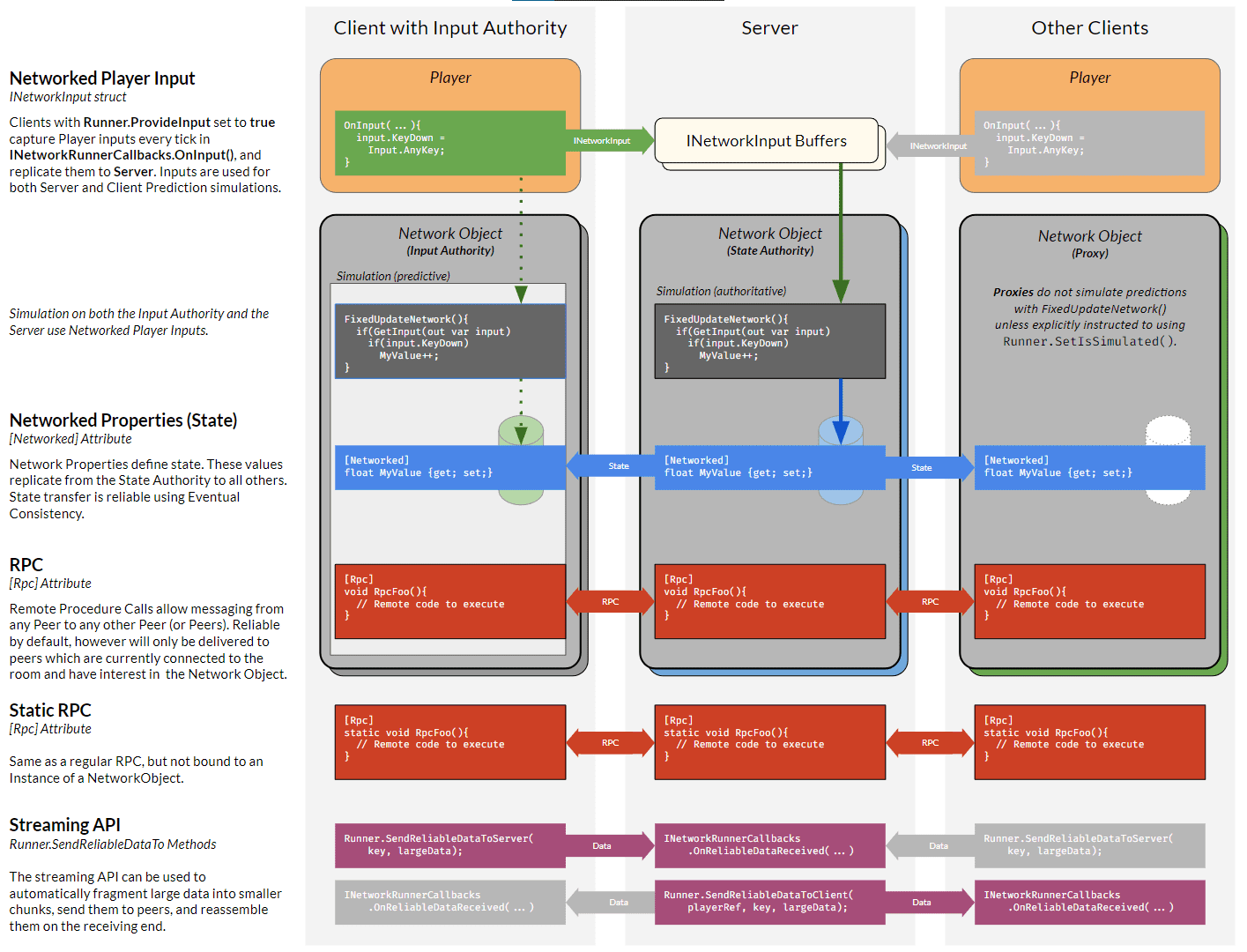
Using a Networked Property in Fusion:
C#
[Networked] public int lives { get; set; }
Defining and Setting Network Input for client-side predicted movement:
C#
public struct NetworkInputData : INetworkInput
{
public Vector3 direction;
}
public class InputPollBehaviour : SimulationBehaviour, INetworkRunnerCallbacks
{
...
public void OnInput(NetworkRunner runner, NetworkInput input)
{
NetworkInputData data = new NetworkInputData();
if (Input.GetKey(KeyCode.LeftArrow))
{
data.direction.x = -1;
}
else if (Input.GetKey(KeyCode.RightArrow))
{
data.direction.x = 1;
}
if (Input.GetKey(KeyCode.DownArrow))
{
data.direction.z = -1;
}
else if (Input.GetKey(KeyCode.UpArrow))
{
data.direction.z = 1;
}
input.Set(data);
}
...
}
Using Network Input for client-side predicted movement:
C#
public override void FixedUpdateNetwork
{
if (GetInput(out NetworkInputData data))
{
data.direction.Normalize(); // normalize to prevent cheating with impossible inputs
_characterController.Move(5 * data.direction * Runner.DeltaTime);
}
}
Declaring a Remote Procedure Call (RPC) in Fusion:
C#
[Rpc(RpcSources.InputAuthority, RpcTargets.StateAuthority)]
public void RPC_Configure(string name, Color color)
{
playerName = name;
playerColor = color;
}
Choosing the Right Mode
Fusion supports three fundamentally different network topologies with the same API as well as a single player mode with no network connection.
The first step when starting with Fusion is to chose which mode to use.
The Quadrant provides a good starting point for deciding what mode is right for your application.
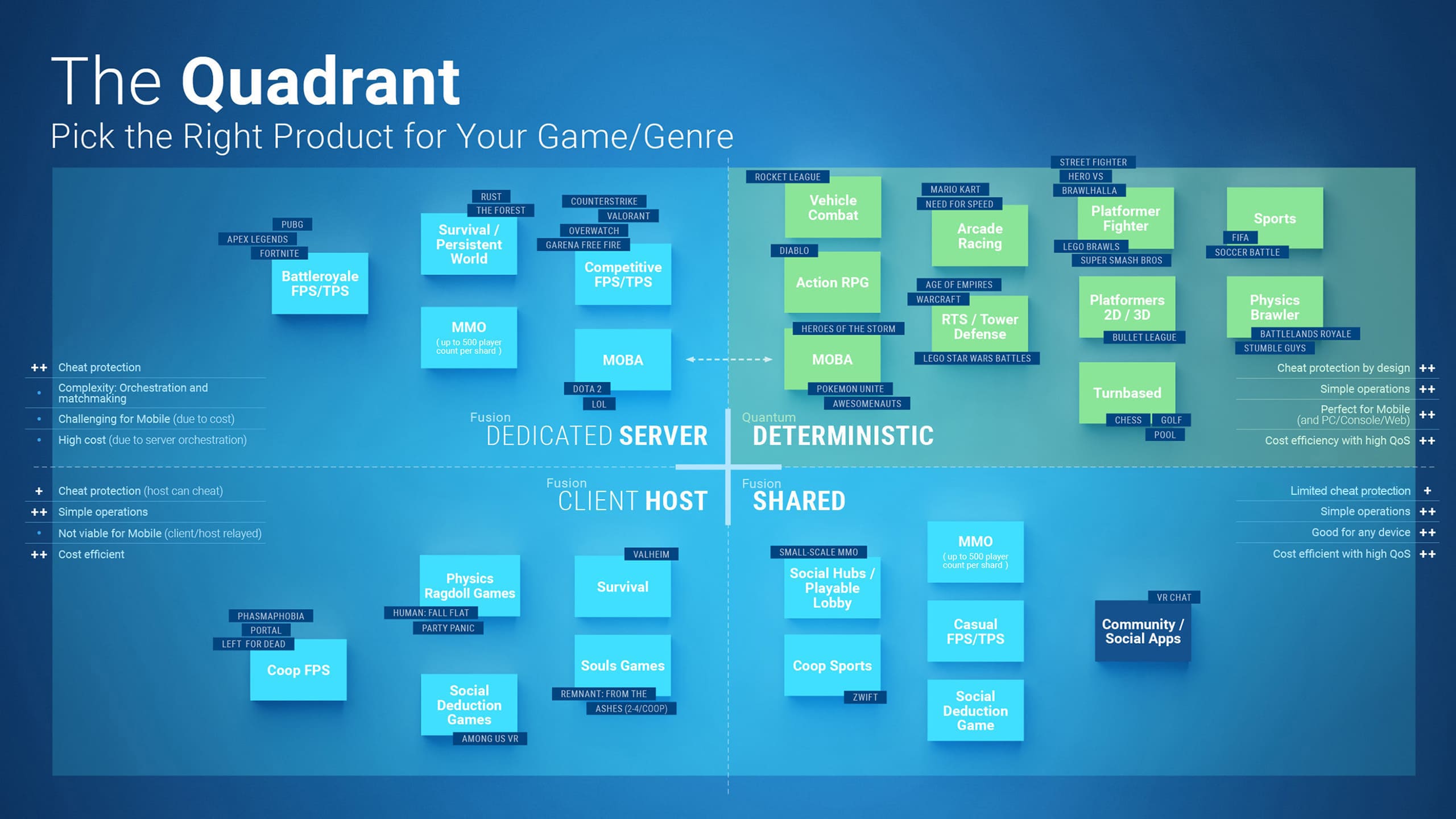
Tutorials
If you already have a good idea for what mode to use you can get started with the tutorials:
If you're not sure yet, keep reading!
Quadrant Comparisons
In the Quadrant, four options are displayed:
- Fusion - Dedicated Server
- Fusion - Client Host
- Fusion - Shared
- Quantum
These are compared over the following categories:
- Suggested Game Types: What type of games work well for this solution?
- Cheat Protection: How easy is it for players to cheat?
- Server Complexity: How complex is it to set up online capabilities for this solution?
- Mobile Quality: How well does this solution work for mobile games and applications?
- Cost Efficiency: How expensive is this solution?
Every project has its own needs, so it's important to compare and understand the differences between these choices and the frameworks available through Photon. Note, this documentation focuses solely on Photon Fusion, so if you want to read more about Photon Quantum, you can do so here.
Suggested Game Types
Some game genres work better for different Fusion solutions than others.
- Fusion - Dedicated Server: competitive games with a lot of users often work well in Server Mode due to their stronger cheat protection. Additionally, because the server has state authority over the game world, ones that require a persistence are good for this solution as well.
- Fusion - Client Host: Because of the more direct connection to the host server, games that utilize a lot of physics work well for this solution. Additionally, games with few players within a session (2-4), work well in this solution as well. Additionally, Player Hosted games are an ideal choice when developers prioritize convenience over cheating prevention and prefer not to manage dedicated servers, resulting in lower overall costs.
- Fusion - Shared: This solution is good for more casual games or those with a lot of players, especially applications in which players will enter and leave often since doing so will not disrupt gameplay.
Note, there is some overlap between the suggested game types with each of Fusion's solutions, so it's important to have a knowledgeable understanding of the differences between each of solution to see if it's the best one for the game being developed.
Cheat Protection
Cheating players, capable of ruining any experience for players if left unchecked, are just an unfortunate reality of online gaming. When it comes to cheating, Fusion's solutions have varying levels of protection by default:
- Fusion - Dedicated Server: Cheat protection is strong because the dedicated server has state authority over the game. If a player tries to cheat, the server can validate and prevent or react to a client who is trying to do so. When using
NetworkInput
andNetworkedProperties
for the gameplay cheating that affects the gameplay state such as speed hacks, or adjusting variables via memory modification is impossible. The only possible cheats in a well-designed Server Mode game are aimbots (A program instead of a user provides inputs) or wall-hacks (can be limited with object interest). - Fusion - Client Host: Because the host player also acts as the server, the same cheat protection applies for clients except the host. The host client, can cheat and modify any value and has access to full information.
- Fusion - Shared: Because players have State Authority over objects they control, cheating can occur without extra precautions being taken. This includes modifying any
NetworkedProperty
to any value or sendingRPCs
at any time and more.
Server Complexity
When comparing Fusion's three solutions through this lens, using a Dedicated Server with Photon Fusion is really the only unique solution. Setting up a dedicated server requires the headless server version of the game to run on servers managed by the user in-house or a third party server hosting solution, which requires more setup and costs much more to operate.
Mobile Quality
Mobile games with online multiplayer are popular but can be challenging due to mobile devices' limited capabilities and wireless connection.
- Fusion - Shared: The recommended mode for mobile. Due to Fusion's performance and speed, Shared Mode works well since the connection is to the cloud and not to other players directly. Shared Mode is also the only mode that does not need to run resimulations which in many cases reduces CPU usage.
- Fusion - Dedicated Server: Is expensive to operate due to additional third party hosting costs for the servers. Only recommended for high ARPU mobile titles.
- Fusion - Client Host: Host Mode is not recommended for mobile except for special use-cases. Because one of the players will also be acting as the server, having a poor connection can make this a difficult and unreliable solution for mobile. Mobile games also have the highest player quit rates which when the host quits the game will lead to a short break while migrating to a new host. On Mobile NAT Punchthrough success rates are lower than other platforms (mobile networks don't allow for this) which causes a lot of connections to be relayed over the cloud which adds additional latency.
WebGL Builds
We strongly recommend Shared Mode on WebGL.
Using shared mode on WebGL provides much better latency than Host / Server because a direct connection to the cloud can be established. CPU usage is also much lower which is important due to WebGL running only on a single thread.
Server and Host mode are not recommended and by default disabled.
Though users can technically act as the server in WebGL builds, either through Dedicated Server or Client Host topologies, it is not recommended due to performance and networking limitations. Typically, WebGL runs on a single thread, which is suspended when the page is not in focus (background tab). While this is fine for individual players, suspending the server of a session will affect all players in a match. Additionally, if a WebGL build acts as the server, it will always utilize a relay connection since direct connections are not supported for WebGL builds.
To enable Server / Host Mode on WebGL toggle "Enable ClientServer Modes in WebGL builds" in the NetworkProjectConfig
to true.
Cost Efficiency
The cost of using Photon solutions is comprised of two factors: concurrent users (CCU) and bandwidth. CCU for all three Fusion solutions are the same. Bandwidth costs is dependant upon how much information is being sent over the network by users. When using Shared Mode or Host mode with a relay server, bandwidth use becomes more of a factor to keep in mind although a well optimized Fusion game should not reach the free bandwidth limit. When using Server Mode, bandwidth pricing will depend more on the third party server provider. You can read more about the specifics of Fusion's pricing here.
Topology Differences
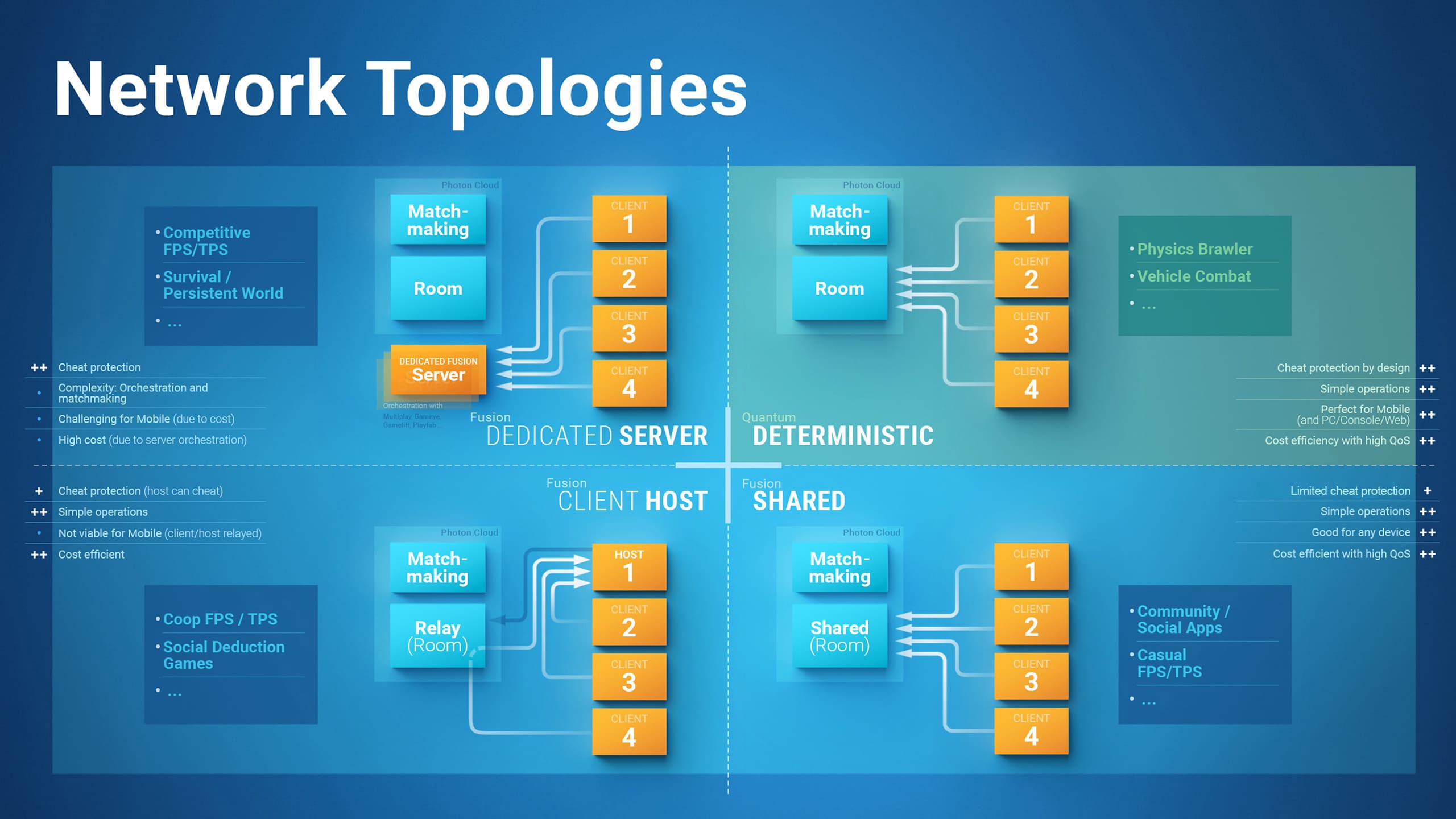
Server Mode
In Server Mode the server has full and exclusive State Authority over all objects, no exceptions.
Clients can only modify networked objects by sending their input to the server (and having the server react to that input) or by requesting a change using an RPC.
The server application is built from the Unity project and runs a full headless Unity build. This headless build needs to be hosted on a server machine or a cloud hosted server. Photon does not provide servers for hosting a dedicated Fusion server application.
Client Side Prediction
Client Side Prediction is a popular multiplayer architecture in which clients use their inputs to predict their own movement while awaiting confirmation from the server. This hides latency and allows the gameplay to feel snappy.
In Fusion's Server Mode, any changes a client makes directly to the networked state are only a local prediction, which will be overridden with actual authoritative snapshots from the server once those are received. This is known as reconciliation, as the client is rolled back to the server-provided state and re-simulated forward to the local (predicted) tick.
If previous predictions were accurate, this process is seamless. If not, the state will be updated, and because the network state is separate from the rendering state, the rendering may either snap to this new state or use various forms of interpolation, error correction and smoothing to reduce the visual artifacts caused by the correction.
Host Mode
In Host Mode, the host acts as both a server and a client. The host has a local player, polls input for it and interpolates the rendering as expected of a client.
Overall, this mode is equivalent to the Server Mode but is much cheaper to run as no dedicated server hosting costs are incurred. However, this comes at the price of the state authority's trustworthiness—in other words, a rogue host can cheat.
When running hosted mode from behind a firewall or a router, the Photon cloud transparently provides UDP punch through or package relay as needed.
Since the session is owned by the host, it will be lost if the host disconnects. Fusion does provide a host migration mechanism to allow transferring of state authority to a new client in situations like this, however this is not automatic in Host Mode and requires special handling in the client code.
Shared Mode
In Shared Mode, authority over network objects is distributed among all clients. Specifically, each client initially has State Authority over objects they spawn, but are free to release that State Authority to other clients. Optionally, clients may be allowed to take State Authority at will.
Features such as client side prediction and rollback are not available in this mode. The simulation always moves forward at the same tick rate on all clients.
The Shared Mode network session is owned by the Photon cloud and remains alive as long as any client is connected to it. Even if the Master Client disconnects, a new Master Client is assigned, transferring NetworkObjects the previous MasterClient had State Authority over without disrupting gameplay. It should be noted that this is not the same as the host migration mechanism previously mentioned.
For those coming from Photon Unity Networking (PUN), Shared Mode is similar in many ways, albeit more feature complete, faster, and with no run-time allocation overhead.
Back to top