Webhooks
Overview
Webhooks are primarily used to have a trusted source of game configurations and room compositions and can greatly contribute to the safety of an online application.
The default cloud plugin has support for different hooks defined via the AppID Dashboard.
Once configured and enabled, the Photon Cloud will send WebRequests (HTTP POST
) to a custom backend and will use the response data (Json) for various configurations on the Photon rooms and game sessions.
Setup
Webhooks are enabled per AppId on the Photon Dashboard.
- Navigate to the Photon Dashboard and log in.
- Find the AppId and click
Manage
. - Scroll down to Plugins and click
Edit
. - Click
Add New Pair
and addkeys
andvalues
(the maximum length allowed for each setting value is 1024 chars). - Press
Save
and wait up to one minute for changes to take effect.
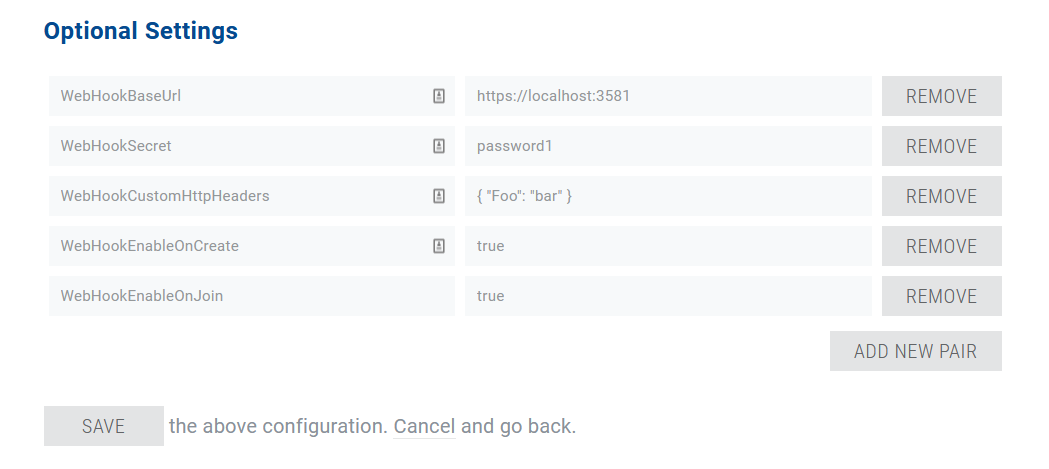
Dashboard Configurations
Key | Type | Example | Description |
---|---|---|---|
WebHookBaseUrl |
string |
https://localhost:3581 |
The base url of the custom backend. Webhook paths will be appended, for example: {WebHookBaseUrl}/game/create Must always be set. |
WebHookIntegration |
string |
Default |
The selected webhook integration (default is Default ).Default , PlayFab |
WebHookSecret |
string |
********** |
This will be send with each web request and can be used to authenticate the request. |
WebHookCustomHttpHeaders |
Dictionary <string, string> |
{"A": "Foo", "B": "100" } |
A JSON dictionary. All entries will be added to the custom web request headers. |
WebHookEnableOnCreate |
bool |
true |
If set to true , the CreateGame webhook will be fired when a client is creating a Game Session. |
WebHookEnableOnClose |
bool |
false |
If set to true , the CloseGame webhook will be fired when the Game Session was closed. |
WebHookEnableOnJoin |
bool |
true |
If set to true , the JoinGame webhook will be fired when any client tries to join a Game Session. |
WebHookEnableOnLeave |
bool |
false |
If set to true , the LeaveGame webhook will be fired when any client leaves a Game Session |
Webhook API
Webhooks send Json content and only accept Json content as a response. UTF-8
charset for Json is mandatory.
Webhooks expect HTTP response codes 200
or 400
:
200
: successful request.400
: error or operation has been denied (e.g. a client cannot create a room/game session).
The Photon server retries webhooks after transportation errors three times as well as after receiving StatusCode 503
(Service Unavailable) with delay of 400ms, 1600ms and 6400ms. The timeout before the request fails and is not retried is 10 seconds.
Fill out the WebhookError definition to return information of the nature of an error back to the Photon plugin. It can be used for:
- Logging;
- Returning information to the client;
- A custom plugin to add further custom error handling;
Common Request Headers
These common request headers are added to every web request.
Name | Type | Content | Description |
---|---|---|---|
Accept |
string |
application/json |
Webhooks only accept JSON as a response body |
Accept-Charset |
string |
utf-8 |
Webhooks only accept utf-8 as response body charset |
Content-Type |
string |
application/json |
Webhooks all send JSON body data |
X-SecretKey |
string |
********** |
This key should only be know to the custom backend and should be used to authenticate the incoming web request. This is set on the Photon Dashboard as WebHookSecret . |
X-Origin |
string |
Photon |
Will always be set to "Photon" |
CreateGame
This webhook is called before the room/game session is created on the Photon Server. The creation is blocked until the webhook receives a response, which will affect the time clients require to create a connection.
A CreateGame
webhook is always a JoinGame
request for the user that initiates the room/game session creation. There will be no subsequent JoinGame
webhook for this user. This webhook shares the data from the JoinGame
webhook.
Requires WebHookBaseUrl
and WebHookEnableCreateGame
to be set on the Photon dashboard.
JavaScript
POST https://{WebHookBaseUrl}/game/create
CreateGame Request
Name | Type | Example | Description |
---|---|---|---|
AppId |
string |
d1f67eec-51fb-45c1 |
The Photon AppId. |
AppVersion |
string |
1.0-live |
The AppVersion used when creating the room/game session. |
Region |
string |
eu |
The Region code of the Game Server that the room/game session was created in. |
Cloud |
string |
1 |
The Cloud Id of that the Game Server is running on. |
UserId |
string |
db757806-8570-45aa |
The UserId of the client that creates the room/game session. |
RoomName |
string |
e472a861-a1e2-49f7 |
The room/game session Name. |
GameId |
string |
0:eu:e472a861-a1e2-49f7 |
A unique GameId which is composed of {Cloud:}{Region:}RoomName . Can be overwritten in the response. |
EnterRoomParams |
EnterRoomParams |
JSON: See EnterRoomParam section |
The Photon room/game session Options sent by the client. |
Json Example:
JSON
{
"AppId": "d1f67eec-51fb-45c1",
"AppVersion": "1.0-live",
"Region": "eu",
"Cloud": "1",
"UserId": "db757806-8570-45aa",
"RoomName": "e472a861-a1e2-49f7",
"GameId": "0:eu:e472a861-a1e2-49f7",
"EnterRoomParams": {
"RoomOptions": {
"IsVisible": true,
"IsOpen": true
}
}
}
HTTP Response Codes
Name | Type | Description |
---|---|---|
200 OK |
CreateGame Response |
room/game session creation can commence, config data from the response will overwrite data sent by the client. |
400 Bad Request |
WebhookError |
room/game session creation is not allowed and will be canceled. The client will receive an error. |
CreateGame Response
Name | Type | Description |
---|---|---|
GameId |
string |
Overwrites the GameId used in subsequent web requests. Can be null or omitted. |
EnterRoomParams |
EnterRoomParams |
Enforce selected Room Options during its creation. The JSON object does not have to include all members, only the ones that should be overwritten.Only the initial options are protected by sending EnterRoomParams . Most of them can be changed by clients sending Photon Room properties. To block this enable the Photon dashboard property BlockRoomProperties . Can be null or omitted. |
Json Example:
JSON
{
"GameId": "0:eu:db757806-8570-45aa",
"EnterRoomParams": {
"RoomOptions": {
"CustomRoomProperties": {
"GameType": "CUSTOM_GAME_TYPE",
"CustomData": 101
}
}
}
}
JoinGame
The JoinGame
webhook is send before a client joins an existing room/game session. Return 200
to allow or 400
to cancel the joining.
Requires WebHookBaseUrl
and WebHookEnableOnJoin
to be set on the Photon AppId Dashboard.
JavaScript
POST https://{WebHookBaseUrl}/game/join
JoinGame Request
Name | Type | Example | Description |
---|---|---|---|
AppId |
string |
d1f67eec-51fb-45c1 |
The Photon AppId |
GameId |
string |
0:eu:db757806-8570-45aa |
Unique GameId |
UserId |
string |
db757806-8570-45aa |
Photon UserId |
Json Example:
JSON
{
"GameId": "0:eu:db757806-8570-45aa",
"UserId": "db757806-8570-45aa"
}
HTTP Response Codes
Name | Type | Description |
---|---|---|
200 OK |
JoinGame Response |
The client will join the room. |
400 Bad Request |
WebhookError |
Joining the room will fail. |
JoinGame Response
Json Example:
JSON
{
// empty
}
LeaveGame
The LeaveGame
webhook is send after the client leaves an existing room/game session.
Requires WebHookBaseUrl
and WebHookEnableOnLeave
to be set on the Photon AppId Dashboard.
JavaScript
POST https://{WebHookBaseUrl}/game/leave
LeaveGame Request
Name | Type | Example | Description |
---|---|---|---|
AppId |
string |
d1f67eec-51fb-45c1 |
The Photon AppId |
GameId |
string |
0:eu:db757806-8570-45aa |
Unique GameId |
UserId |
string |
db757806-8570-45aa |
Photon UserId |
Json Example:
JSON
{
"GameId": "0:eu:db757806-8570-45aa",
"UserId": "db757806-8570-45aa"
}
HTTP Response Codes
Name | Type | Description |
---|---|---|
200 OK |
LeaveGame Response |
Just a confirmation of receipt. |
400 Bad Request |
WebhookError |
Error is ignored, it will just be logged on the Photon Cloud. |
LeaveGame Response
Json Example:
JSON
{
// empty
}
CloseGame
The CloseGame
webhook is sent when the room/game session is closed after all clients have left.
Requires WebHookBaseUrl
and WebHookEnableOnClose
to be set on the Photon dashboard.
JavaScript
POST https://{WebHookBaseUrl}/game/close
CloseGame Request
Name | Type | Example | Description |
---|---|---|---|
AppId |
string |
d1f67eec-51fb-45c1 |
The Photon AppId |
GameId |
string |
0:eu:db757806-8570-45aa |
Unique game id |
CloseReason |
int (CloseReason ) |
0 |
The reason why this room/session has been closed. |
Json Example:
JSON
{
"GameId": "0:eu:db757806-8570-45aa",
"CloseReason": 0
}
HTTP Response Codes
Name | Type | Description |
---|---|---|
200 OK |
Confirmation of receipt. |
CloseReason
Name | Value | Description |
---|---|---|
Ok |
0 |
Session was closed without errors. |
FailedOnCreate |
1 |
Session was closed because it failed to Create. |
Name | Value | Description | ExceptionOnUpdateLoop |
101 |
Any exception occurred on the Plugin Server Update Loop, causing the Session to be closed. |
---|---|---|
ExceptionOnHostMigration |
102 |
Any exception while performing the Host Migration on the Plugin side. |
ServerHasDisconnected |
103 |
Fusion Server has disconnected from the Session, all clients must be disconnected. |
FailedToSetupServer |
104 |
Any exception while performing the Plugin Server Setup, causing the Session to be closed. |
FailedToParseNetworkProjectConfig |
105 |
Failed to parse the Fusion's NetworkProjectConfig . |
EnterRoomParams
This definitions is designed to be similar to the EnterRoomParams
class in Photon Realtime. It includes all options that can be set by the CreateGame
webhook. When composing the Json Response every member is optional and can be null or not set.
Name | Type | Description |
---|---|---|
RoomOptions |
RoomOptions |
RoomOptions object |
ExpectedUsers |
string[] |
A list of UserIds that are permitted to enter the room/session (additionally to the user creating the room). If MaxPlayers is greater than the number ExpectedUsers listed, any player may join and fill the unreserved slots.Works only for RoomJoin() not JoinRandom() . |
Json example:
JSON
{
"RoomOptions": {
"IsVisible": true,
"IsOpen": true,
"MaxPlayers": 8,
"PlayerTtl": null,
"EmptyRoomTtl": 10000,
"CustomRoomProperties": {
"Foo": "bar",
"PlayerClass": 1
},
"CustomRoomPropertiesForLobby": [
"Foo"
],
"SuppressRoomEvents": null,
"SuppressPlayerInfo": null,
"PublishUserId": null,
"DeleteNullProperties": null,
"BroadcastPropsChangeToAll": null,
"CleanupCacheOnLeave": null,
"CheckUserOnJoin": null
},
"ExpectedUsers": [
"A",
"B",
"C"
]
}
Hint
Fusion only supports overriding the RoomOptions.CustomRoomProperties
of a Game Session. All other fields are ignored if returned to the Photon Cloud.
RoomOptions
All values are nullable types and can be set to null
or be omitted when sending back to the Quantum server, in which case this response will not alter the nulled or omitted room property and will remain the default or the value send by the client when creating the room.
Name | Type | Description |
---|---|---|
IsVisible |
bool |
Defines if this room is listed in the Photon matchmaking. |
IsOpen |
bool |
Defines if this room can be joined by other clients. |
MaxPlayers |
byte |
Max number of players that can be in the room at any time. 0 means "no limit". |
PlayerTtl |
int |
Time To Live (TTL) for an 'actor' in a room. If a client disconnects, this actor is inactive first and removed after this timeout. In milliseconds. |
EmptyRoomTtl |
int |
Time To Live (TTL) for a room when the last player leaves. Keeps room in memory for case a player re-joins soon. In milliseconds. |
CustomRoomProperties |
|
The room's custom properties to set during creation. |
CustomRoomPropertiesForLobby |
string[] |
Defines which of the custom room properties get listed in the lobby. Value type of the properties has to be bool , byte , short , int , long or string .Max number of properties is 3. The max length for string value is 64. Key restrictions can also be enforced by the Photon dashboard property: AllowedLobbyProperties . |
SuppressRoomEvents |
bool |
Tells the server to skip room events for joining and leaving players. Default is false . |
SuppressPlayerInfo |
bool |
Disables events join and leave from the server as well as property broadcasts in a room (to minimize traffic). Default is false . |
PublishUserId |
bool |
Defines if the UserIds of players get "published" in the room. Useful for FindFriends, if players want to play another game together. Default is false . |
DeleteNullProperties |
bool |
Optionally, properties get deleted, when null gets assigned as value. Default is false . |
Name | Type | Description |
---|---|---|
CleanupCacheOnLeave |
bool |
Removes a user's events and properties from the room when a user leaves. Should always be true . |
CheckUserOnJoin |
bool |
By default, property changes are sent back to the client that's setting them to avoid de-sync when properties are set concurrently. Should always be true . |
WebhookError
Name | Type | Description |
---|---|---|
Status |
int |
HTTP status code |
Error |
string |
Error name |
Message |
string |
Error message |
Json example:
JSON
{
"Status": 400,
"Error": "PlayerNotAllowed",
"Message": "LoremIpsum"
}
Photon Cloud Web Request Limitations
There are global limitations on the Photon Server per room/session. If they are hit web requests and reponse can be dropped.
HttpRequestTimeout
: 30000LimitHttpResponseMaxSize
: 200000MaxQueuedRequests
: 5000